Dark Nothing..

Posts : 1 Join date : 2011-07-01
 | Subject: Working examples of some random stuff I coded. Fri Jul 01, 2011 11:29 am | |
| Some of these were school assignments, others were random thoughts. Printing Program [Input is taken then prioritized then given back to user. Not literally printed] - start.java:
- Code:
-
import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.image.BufferedImage; import java.io.*; import java.net.URL; import java.util.ArrayList; import java.util.Scanner;
import javax.swing.*;
public class start implements ActionListener {
private static final Printer Printer = null; /** * @author Dean */ public static ArrayList<Printer> printer = new ArrayList<Printer>(); public static ArrayList<Printer> printers = new ArrayList<Printer>(); int speed = 3000; public static void main(String[] args) { String title, body, moreinput = "Yes"; int priority; Scanner input = new Scanner(System.in); for (int i=0; moreinput.charAt(0)== 'y' || moreinput.charAt(0) == 'Y'; i++) { printer.add(new Printer()); if (i>0) { input.nextLine(); } System.out.println("What would you like the title of the document to be?:"); title = input.nextLine(); printer.get(i).setTitle(title); System.out.println("What would you like the body of the document to be?:"); body = input.nextLine(); printer.get(i).setBody(body);
System.out.println("What is the priority of this document?"); priority = input.nextInt(); printer.get(i).setPriority(priority);
System.out.println("Would you like to enter more data?(Yes or No)"); moreinput = input.next(); } //Printing all System.out.println("Now printing your files..."); for (int i=0; i<printer.size(); i++) { int highestpriority = Integer.MIN_VALUE; int savespot = 0; // This is used to make the new priority of the document irrelevent when searching for the next highest priority. printers.add(new Printer()); for (int k=0; k<printer.size(); k++) { if (printer.get(k).getPriority()>highestpriority) { highestpriority = printer.get(k).getPriority(); savespot=k; } if (k==(printer.size()-1)) { printers.get(i).setPriority(highestpriority); printers.get(i).setTitle(printer.get(savespot).getTitle()); printers.get(i).setBody(printer.get(savespot).getBody()); printer.get(savespot).setPriority(Integer.MIN_VALUE); System.out.println("Priority: "+printers.get(i).getPriority()); System.out.println("Title: "+printers.get(i).getTitle()); } } } for (int i=0; i<printers.size(); i++) { //timetoprint.start(); System.out.println("Document Number: "+ (i+1)); System.out.println("Title: "+printers.get(i).getTitle()); System.out.println("Body: "+printers.get(i).getBody()); System.out.println("Priority: "+printers.get(i).getPriority()+"\n"); if (i!=(printers.size()-1)){ // timetoprint.restart(); } } } }
- Printer.java:
- Code:
-
import java.util.ArrayList; import java.util.Arrays;
public class Printer {
String title = ""; String body = ""; int priority = 0; int titlenumber = 0; int bodynumber = 0; int prioritynumber = 0; /* public Printer () {
//title = new String[50]; //body = new String[50]; //priority = new String[50]; this.title = title; this.body = body; this.priority = priority; this.titlenumber = titlenumber; this.bodynumber = bodynumber; this.prioritynumber = prioritynumber; } */ public void setTitle(String senttitle) { //this.title[titlenumber] = senttitle; //titlenumber++; this.title = senttitle; } public String getTitle() { return this.title; } public void setBody(String sentbody) { //this.body[bodynumber] = sentbody; //bodynumber++; this.body = sentbody; } public String getBody() { return this.body; } public void setPriority(int sentpriority) { //this.priority[prioritynumber] = sentpriority; //prioritynumber++; this.priority = sentpriority; } public int getPriority() { return this.priority; } }
Number Input Analyzer (Takes in your data and organizes it) - NumberInputAnalyzerExecutable.java:
- Code:
-
import java.util.Scanner;
public class InputAnalyzerExecutable {
/** * This program will analyze your input and give you the Average, Mode, Min, Max, Range, * and a Histogram to display your data in. This program uses Arrays and multiple classes. * * @author Dean Johnson */ // Defining variables public static void main(String[] args) { Scanner userInput = new Scanner(System.in); InputAnalyzerCode spot = new InputAnalyzerCode(); int sizeOfArray; boolean errorFlag; System.out.println("This program will analyze your input and give you the Average, Mode, Min, Max, Range," + "and a Histogram to display your data in."); System.out.println("How many values will you be entering for the program to analyze?"); sizeOfArray = userInput.nextInt(); int[] numbers = new int [sizeOfArray];// Defining the array. //Assigns a value to every value in the integer for (int i = 0; i < numbers.length; i++) { numbers[i] = 0; } // This loop is for entering a number for each value for (int p = 0; p < numbers.length; p++) { errorFlag = true; String userData = ""; int spotInArray = p+1; System.out.println("\nWhich number would you like analyzed? You are entering number "+spotInArray+". (Hint: Type 'done' to stop entering numbers early): "); userData = userInput.next(); // breaks out of loop, only needed because of being stuck in a loop. and no need to continue input if they are done. if (userData.equals("done")) { break; }// end of if statement to break the loop
// Try and catch, when you test for whether or not the integer is greater than 1 or less than 50 // it returns a NumberFormatException if the users input was a string. try { if (Integer.parseInt(userData.trim()) < 1 || Integer.parseInt(userData.trim()) > 50 ) { errorFlag = true; } else {errorFlag = false;} } // e is user defined catch (NumberFormatException e) { } // e is a user defined variable // Checks for legit user input while (errorFlag == true) { System.out.println("Your either had letters in it or was less than 1 or greater than 50. (Hint: Type 'done' to exit input.)"); // Taking the users data to check for the first letter being a character or not. userData = userInput.next(); // breaks out of loop, only needed because of being stuck in a loop. and no need to continue input if they are done. if (userData.equals("done")) { break; }// breaks while loop // Try and catch, when you test for whether or not the integer is greater than 1 or less than 50 // it returns a NumberFormatException if the users input was a string. try { if (Integer.parseInt(userData.trim()) < 1 || Integer.parseInt(userData.trim()) > 51 ) { errorFlag = true; } else {errorFlag = false;} } catch (NumberFormatException e) {} // e is a user defined variable } // end of while checking for legit entries // breaks out of loop, only needed because of being stuck in a loop. and no need to continue input if they are done. if (userData.equals("done")) { break; }// breaks for loop // Gives numbers the value the user input ` numbers[p] = Integer.parseInt(userData.trim()); }// end of for loop userInput.close(); spot.setUserInput(numbers); // Sends data to be used for finding out Average,Max,Min, etc. spot.setSizeOfArray(sizeOfArray); // Uses this to determine size of array //Output for user System.out.println("Average: "+spot.Average()); System.out.println("Minimum: "+spot.Minimum()); System.out.println("Maxiumum: "+spot.Maximum()); System.out.println(spot.Range()); System.out.println("\n"+spot.Mode()); System.out.println("\nHistogram:"); System.out.print(spot.Histogram()); } /** // This method is used for debugging to show the values in an array public static String valuesInArray () { String valueInArray = ""; for (int i = 0; i < 5; i++) { valueInArray += numbers[i]+", "; } return valueInArray; }// end of valuesInArray class **/ }// end of class
- NumberInputAnalyzerCode.java:
- Code:
-
public class InputAnalyzerCode { private int sizeOfArray, numberOfNumbersInArray; private int[] numbers = new int [sizeOfArray];
public void setUserInput (int[] sentNumbers) { this.numbers = sentNumbers; } public void setSizeOfArray (int sizeArray) { this.sizeOfArray = sizeArray; } public double Average () { double average = 0, numbersAdd = 0; int numberOfNumbersInArray = 0; for (int i = 0; i < numbers.length; i++) { numbersAdd += numbers[i]; if (numbers[i] != 0) { // if the number is not 0 (initialized the array as 0, add the number to divide by numberOfNumbersInArray++; }// end of if adding the number of times to be divided }// end of for loop doing calculations average = numbersAdd / numberOfNumbersInArray; return average; }// end of getting Average public int Maximum () { int max = -2147000000; for (int c = 0; c < numbers.length; c++) { if (numbers[c] > max) { max = numbers[c]; }// end of the if statement to determine the maxiumum }// end of c, checking each value inside of the array return max; }// end of getting Maximum number public int Minimum () { int min = 2147000000; for (int c = 0; c < numbers.length; c++) { if (numbers[c] < min && numbers[c] > 0) { min = numbers[c]; }// end of the if statement to determine the minimum }// end of c, checking each value inside of the array return min; }// end of getting Minimum number public String Range () { String Range = "Range: "+Minimum()+" - "+Maximum(); return Range; }// end of method that finds the Range public String Mode () { String Mode = ""; int[] amountOfNumbers = new int [sizeOfArray]; int mostOccuring = 0, modeOfArray = 0; for (int i = 0; i < numbers.length; i++) { for (int c = 1;c < sizeOfArray; c++) { if (numbers[i] == numbers[c]) { amountOfNumbers[c]++; }// end of the if statement for mode }// end of second loop }// end of first loop for (int p = 0; p < amountOfNumbers.length; p++) { if (amountOfNumbers[p] > mostOccuring) { mostOccuring = amountOfNumbers[p]; // Gives the amount of times a number was input to highestOccurance modeOfArray = numbers[p]; }// end of if giving mode values }// end of finding mode Mode = "Mode: "+modeOfArray+". It occurs "+mostOccuring+" times."; return Mode; }// end of method Mode public String Histogram () { String Histogram = "", fives = "1-5: ", tens = "\n6-10: ", fifteens = "\n11-15: ", twentys = "\n16-20: ", twentyfives = "\n21-25: "; String thirtys = "\n26-30: ", thirtyfives = "\n31-35: ", fortys = "\n36-40: ", fortyfives = "\n41-45: ", fiftys = "\n46-50: "; for (int p = 0; p < numbers.length; p++) { // Checks the values of each number in the array /** * These statements will allow each number to be placed into a category which will later * be added with all other categorys to form the histogram */ // if (numbers[p] > 0 && numbers[p] < 6) { fives += numbers[p]+" "; } else if (numbers[p] > 5 && numbers[p] < 11) { tens += numbers[p]+" "; } else if (numbers[p] > 10 && numbers[p] < 16) { fifteens += numbers[p]+" "; } else if (numbers[p] > 15 && numbers[p] < 21) { twentys += numbers[p]+" "; } else if (numbers[p] > 20 && numbers[p] < 26) { twentyfives += numbers[p]+" "; } else if (numbers[p] > 25 && numbers[p] < 31) { thirtys += numbers[p]+" "; } else if (numbers[p] > 30 && numbers[p] < 36) { thirtyfives += numbers[p]+" "; } else if (numbers[p] > 35 && numbers[p] < 41) { fortys += numbers[p]+" "; } else if (numbers[p] > 40 && numbers[p] < 46) { fortyfives += numbers[p]+" "; } else if (numbers[p] > 45 && numbers[p] < 51) { fiftys += numbers[p]+" "; } }// End of the for loop for checking each number individually Histogram = fives + tens + fifteens + twentys + twentyfives + thirtys + thirtyfives + fortys + fortyfives+ fiftys; return Histogram; } }// end of the class
TicTacToe [Gotta replace X and O images in the folder that game is compiled to] - TicTacToe.java:
- Code:
-
// GUI imports import javax.swing.*; import java.awt.*; import java.awt.event.*;
public class TicTacToe implements ActionListener{ // Defining Variables static String[] board = new String [9]; // Array and strings that are private to this class JFrame frame; JPanel panel; JLabel Text; JButton topLeft_1, topMid_2, topRight_3, midLeft_4, midMid_5, midRight_6, botLeft_7, botMid_8, botRight_9 ; ImageIcon imgO = createImageIcon("O_tictac.jpg"); ImageIcon imgX = createImageIcon("X_tictac.jpg"); String playerTurn = "X", squaresUsed = ""; int playerX = 112, playerO = 111; static int squaresLeft = 9; // Everything involved in the GUI (Labels, buttons, etc.) public ticTacToe () { // Creates the frame frame = new JFrame("Tic Tac Toe!"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Creates the panel panel = new JPanel(); panel.setLayout(new GridLayout(4, 3)); panel.setBorder(BorderFactory.createEmptyBorder(10,10,10,10)); // Buttons to use for the game. Buttons 1-9 topLeft_1 = new JButton(""); topLeft_1.setActionCommand("topLeft_1"); topLeft_1.addActionListener(this); panel.add(topLeft_1); topMid_2 = new JButton(""); topMid_2.setActionCommand("topMid_2"); topMid_2.addActionListener(this); panel.add(topMid_2); topRight_3 = new JButton(""); topRight_3.setActionCommand("topRight_3"); topRight_3.addActionListener(this); panel.add(topRight_3); midLeft_4 = new JButton(""); midLeft_4.setActionCommand("midLeft_4"); midLeft_4.addActionListener(this); panel.add(midLeft_4); midMid_5 = new JButton(""); midMid_5.setActionCommand("midMid_5"); midMid_5.addActionListener(this); panel.add(midMid_5); midRight_6 = new JButton(""); midRight_6.setActionCommand("midRight_6"); midRight_6.addActionListener(this); panel.add(midRight_6); botLeft_7 = new JButton(""); botLeft_7.setActionCommand("botLeft_7"); botLeft_7.addActionListener(this); panel.add(botLeft_7); botMid_8 = new JButton(""); botMid_8.setActionCommand("botMid_8"); botMid_8.addActionListener(this); panel.add(botMid_8); botRight_9 = new JButton(""); botRight_9.setActionCommand("botRight_9"); botRight_9.addActionListener(this); panel.add(botRight_9); // Label that will announce a winner Text = new JLabel(""); panel.add(Text); // Adds the panel frame.setContentPane(panel); // Sets the frame size and turns it visible //frame.setSize(500,500); frame.setSize(600,300); frame.setVisible(true); } // end of development of GUI public void actionPerformed(ActionEvent event) { boolean checkForSquaresLeft = false; String eventName = event.getActionCommand(); if (checkBoard()) { if (playerTurn.equals("X")) { Text.setText("The winner is Player O!"); } else if (playerTurn.equals("O")) { Text.setText("The winner is Player X!"); } } else if (squaresLeft == 0) { Text.setText("Cats Game!"); }
else if (!checkBoard() || squaresLeft != 0) { if (!squaresUsed.contains(eventName)) { if (eventName.equals("topLeft_1")) { if (playerTurn == "O") { topLeft_1.setIcon(imgO); Text.setText(playerTurn+" Just went"); playerTurn = "X"; board[0] = "O"; } else if (playerTurn == "X") { topLeft_1.setIcon(imgX); Text.setText(playerTurn+" Just went"); playerTurn = "O"; board[0] = "X"; } checkForSquaresLeft = true; squaresUsed += eventName; } // end of button 1 } else if (squaresUsed.contains(eventName) && eventName.equals("topLeft_1")) { Text.setText(""+squaresLeft); } if (!squaresUsed.contains(eventName)) { if (eventName.equals("topMid_2")) { if (playerTurn == "O") { topMid_2.setIcon(imgO); Text.setText(playerTurn+" Just went"); playerTurn = "X"; board[1] = "O"; } else if (playerTurn == "X") { topMid_2.setIcon(imgX); Text.setText(playerTurn+" Just went"); playerTurn = "O"; board[1] = "X"; } squaresUsed += eventName; checkForSquaresLeft = true; } // end of button 1 } else if (squaresUsed.contains(eventName) && eventName.equals("topMid_2")) { Text.setText(""+squaresLeft); } if (!squaresUsed.contains(eventName)) { if (eventName.equals("topRight_3")) { if (playerTurn == "O") { topRight_3.setIcon(imgO); Text.setText(playerTurn+" Just went"); playerTurn = "X"; board[2] = "O"; } else if (playerTurn == "X") { topRight_3.setIcon(imgX); Text.setText(playerTurn+" Just went"); playerTurn = "O"; board[2] = "X"; } squaresUsed += eventName; checkForSquaresLeft = true; } // end of button 1 } else if (squaresUsed.contains(eventName) && eventName.equals("topRight_3")) { Text.setText(""+squaresLeft); } if (!squaresUsed.contains(eventName)) { if (eventName.equals("midLeft_4")) { if (playerTurn == "O") { midLeft_4.setIcon(imgO); Text.setText(playerTurn+" Just went"); playerTurn = "X"; board[3] = "O"; } else if (playerTurn == "X") { midLeft_4.setIcon(imgX); Text.setText(playerTurn+" Just went"); playerTurn = "O"; board[3] = "X"; } squaresUsed += eventName; checkForSquaresLeft = true; } // end of button 1 } else if (squaresUsed.contains(eventName) && eventName.equals("midLeft_4")) { Text.setText(""+squaresLeft); } if (!squaresUsed.contains(eventName)) { if (eventName.equals("midMid_5")) { if (playerTurn == "O") { midMid_5.setIcon(imgO); Text.setText(playerTurn+" Just went"); playerTurn = "X"; board[4] = "O"; } else if (playerTurn == "X") { midMid_5.setIcon(imgX); Text.setText(playerTurn+" Just went"); playerTurn = "O"; board[4] = "X"; } squaresUsed += eventName; checkForSquaresLeft = true; } // end of button 1 } else if (squaresUsed.contains(eventName) && eventName.equals("midMid_5")) { Text.setText(""+squaresLeft); } if (!squaresUsed.contains(eventName)) { if (eventName.equals("midRight_6")) { if (playerTurn == "O") { midRight_6.setIcon(imgO); Text.setText(playerTurn+" Just went"); playerTurn = "X"; board[5] = "O"; } else if (playerTurn == "X") { midRight_6.setIcon(imgX); Text.setText(playerTurn+" Just went"); playerTurn = "O"; board[5] = "X"; } squaresUsed += eventName; checkForSquaresLeft = true; } // end of button 6 } else if (squaresUsed.contains(eventName) && eventName.equals("midright_6")) { Text.setText(""+squaresLeft); } if (!squaresUsed.contains(eventName)) { if (eventName.equals("botLeft_7")) { if (playerTurn == "O") { botLeft_7.setIcon(imgO); Text.setText(playerTurn+" Just went"); playerTurn = "X"; board[6] = "O"; } else if (playerTurn == "X") { botLeft_7.setIcon(imgX); Text.setText(playerTurn+" Just went"); playerTurn = "O"; board[6] = "X"; } squaresUsed += eventName; checkForSquaresLeft = true; } // end of button 1 } else if (squaresUsed.contains(eventName) && eventName.equals("botLeft_7")) { Text.setText(""+squaresLeft); } if (!squaresUsed.contains(eventName)) { if (eventName.equals("botMid_8")) { if (playerTurn == "O") { botMid_8.setIcon(imgO); Text.setText(playerTurn+" Just went"); playerTurn = "X"; board[7] = "O"; } else if (playerTurn == "X") { botMid_8.setIcon(imgX); Text.setText(playerTurn+" Just went"); playerTurn = "O"; board[7] = "X"; } squaresUsed += eventName; checkForSquaresLeft = true; } // end of button 8 } else if (squaresUsed.contains(eventName) && eventName.equals("botMid_8")) { Text.setText(""+squaresLeft); } if (!squaresUsed.contains(eventName)) { if (eventName.equals("botRight_9")) { if (playerTurn.equals("O")) { botRight_9.setIcon(imgO); Text.setText(playerTurn+" Just went"); playerTurn = "X"; board[8] = "O"; } else if (playerTurn.equals("X")) { botRight_9.setIcon(imgX); Text.setText(playerTurn+" Just went"); playerTurn = "O"; board[8] = "X"; } squaresUsed += eventName; checkForSquaresLeft = true; } // end of button 9 } else if (squaresUsed.contains(eventName) && eventName.equals("botRight_9")) { Text.setText(""+squaresLeft); } } // This will clarify the players of the ending of the game. if (checkForSquaresLeft) {squaresLeft--;} // end of the if statement making sure there is still no winner. } // End of actionPerformed private static ImageIcon createImageIcon(String image) { java.net.URL imgURL = ticTacToe.class.getResource(image); if (imgURL != null) {return new ImageIcon (imgURL,"");} else {System.out.println("no picture"); return null;} } // end of the method to create icons private static void runGUI() { ticTacToe test = new ticTacToe(); // Creates a new object for PictureViewer }// end of the runGUI function public static void main(String[] args) { // Main function used to execute the GUI // Methods that create and show the gui javax.swing.SwingUtilities.invokeLater(new Runnable() { public void run() { runGUI(); // runs the gui } // end of run() }); // end of the javax.swing.SwingUtilities.invokeLater(new Runnable() }// end of main method /** public static void printBoard () { System.out.println(" | | "); System.out.println("_"+board[0]+"__|_"+board[1]+"__|_"+board[2]+"__"); System.out.println(" | | "); System.out.println("_"+board[3]+"__|_"+board[4]+"__|_"+board[5]+"__"); System.out.println(" | | "); System.out.println(" "+board[6]+" | "+board[7]+" | "+board[8]+" ");
} **/ public static boolean checkBoard () { // checks for a winner or the board being full boolean pass = false;
if (board[0] == "X" && board[1] == "X" && board[2] == "X") {pass = true;} if (board[3] == "X" && board[4] == "X" && board[5] == "X") {pass = true;} if (board[6] == "X" && board[7] == "X" && board[8] == "X") {pass = true;} if (board[0] == "X" && board[3] == "X" && board[6] == "X") {pass = true;} if (board[1] == "X" && board[4] == "X" && board[7] == "X") {pass = true;} if (board[2] == "X" && board[5] == "X" && board[8] == "X") {pass = true;} if (board[0] == "X" && board[4] == "X" && board[8] == "X") {pass = true;} if (board[2] == "X" && board[4] == "X" && board[6] == "X") {pass = true;} // End of checking O's win
if (board[0] == "O" && board[1] == "O" && board[2] == "O") {pass = true;} if (board[3] == "O" && board[4] == "O" && board[5] == "O") {pass = true;} if (board[6] == "O" && board[7] == "O" && board[8] == "O") {pass = true;} if (board[0] == "O" && board[3] == "O" && board[6] == "O") {pass = true;} if (board[1] == "O" && board[4] == "O" && board[7] == "O") {pass = true;} if (board[2] == "O" && board[5] == "O" && board[8] == "O") {pass = true;} if (board[0] == "O" && board[4] == "O" && board[8] == "O") {pass = true;} if (board[2] == "O" && board[4] == "O" && board[6] == "O") {pass = true;} // End of checking O's win return pass; } // end of checkBoard() }
// This is for reference to how the program USED to work. /** public static void main (String[] args) {
Scanner input = new Scanner(System.in); // Scanner for user input boolean isWinner; char winner = 'a'; // Who the winner is. int playerX = 112, playerO = 111; for (int c = 0; c < board.length; c++) { board[c] = ""+c; } while (!checkBoard()) { // Start of Player Xs turn. System.out.println("Please choose which square you would like to play in Player x."); playerX = input.nextInt(); while (numbers.contains(""+playerX)) { System.out.println("I'm sorry, this square is occupied by Player X or yourself! Please choose another."); playerX = input.nextInt(); board[playerX] = "x"; }// end of while checking for a duplicate number board[playerX] = "x"; numbersX += playerX; numbers += numbersX + numbersO; squaresLeft--; if (checkBoard()){isWinner = true; winner = 'x'; break;} // Start of Player Os turn. System.out.println("Which square would you like to play in Player O?"); playerO = input.nextInt(); while (numbers.contains(""+playerO)) { System.out.println("I'm sorry, this square is occupied by Player X or yourself! Please choose another."); playerO = input.nextInt(); board[playerO] = "o"; }// end of while checking for a duplicate number board[playerO] = "o"; numbersO += playerO; numbers = numbersX + numbersO; squaresLeft--; if (checkBoard()){ isWinner = true; winner = 'O'; break;}
}// end of while for playing game
//I need 2 users, x and y. //I need them to type in a number to give board, and make sure it TAKES the number //so it is unable to be used again.
if (isWinner = true) { System.out.println("Congratulations "+winner+"! You have won the game!"); } else {System.out.println("Cats game.");} }// end of main **/
More coming soon... | |
|
Sorrow Admin
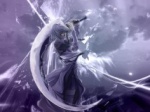
Posts : 9 Join date : 2011-03-28 Age : 34 Location : Burning Emblem
 | Subject: Re: Working examples of some random stuff I coded. Fri Jul 01, 2011 11:44 am | |
| | |
|